Angular2 Routing教程
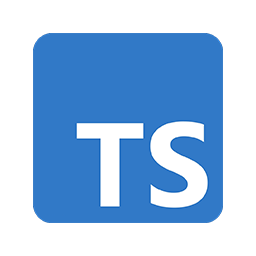
摘要
在Angular应用程序中,如何确定当前活动的路由?
内容
在Angular应用程序中,我们经常需要知道当前活动的路由,以便在导航链接或按钮上添加活动状态的类。在不同版本的Angular中,有多种方法可以实现这一目标。本教程将介绍一些常用的方法。
标记导航链接为活动状态
在Angular中,我们可以使用[routerLinkActive]
指令来将导航链接标记为活动状态。通过给[routerLinkActive]
指令添加一个用于活动状态的CSS类名,当链接的路由与当前路由匹配时,该类名将被添加到链接上。
下面是一个带有活动状态的导航链接的示例:
1- <a [routerLink]="['/home']" [routerLinkActive]="['is-active']">Home</a>
或者,如果只需要一个类,可以简化为:
1- <a [routerLink]="['/home']" [routerLinkActive]="'is-active'">Home</a>
或者,如果只需要一个类,还可以进一步简化为:
1- <a [routerLink]="['/home']" routerLinkActive="is-active">Home</a>
有关更多信息,请参见routerLinkActive指令的文档。
通过注入Location对象来检测当前路由
在Angular中,我们还可以通过注入Location对象来检测当前路由。Location对象提供了一种方便的方式,通过调用path()
方法获取当前路由的路径。
下面是使用Location对象检测当前活动路由的示例:
1import { Location } from '@angular/common';
2
3class MyController {
4 constructor(private location: Location) {}
5
6 // 获取当前路径
7 getCurrentRoute() {
8 return this.location.path();
9 }
10}
请确保首先导入Location对象:
1import { Location } from '@angular/common';
注意,无论使用哪种LocationStrategy
,Location
类都会返回一个规范化的路径,而不会返回具体的路径片段。
参考答案
以下是一些不同版本的Angular中确定活动路由的参考答案:
1- With the new Angular router, you can add a `[routerLinkActive]="['your-class-name']"` attribute to all your links. For example:
2
3```typescript
4<a [routerLink]="['/home']" [routerLinkActive]="['is-active']">Home</a>
You can also use the simplified non-array format if only one class is needed:
1<a [routerLink]="['/home']" [routerLinkActive]="'is-active'">Home</a>
- You can also check the current route by injecting the
Router
object into your controller and using theisActive()
method. For example:
1import { Router } from '@angular/router';
2
3class MyController {
4 constructor(private router: Router) {}
5
6 isActive(route: string) {
7 return this.router.isActive(route, false);
8 }
9}
Or, if you want to check the current URL instead of a specific route:
1import { Router } from '@angular/router';
2
3class MyController {
4 constructor(private router: Router) {}
5
6 isActive(url: string) {
7 return this.router.url === url;
8 }
9}
- You can also use the
routerLinkActive
directive with a template variable. For example:
1<a [routerLink]="'/home'" routerLinkActive #rla="routerLinkActive" [ngClass]="rla.isActive ? 'active' : 'inactive'"></a>
This way, you can check the isActive
property of the routerLinkActive
directive and add the corresponding class to the element.
- Another way is to add the
routerLinkActive
directive to the parent element of the link where you want to apply the active class. For example:
1<ul>
2 <li [routerLinkActive]="['active']"><a [routerLink]="['/home']">Home</a></li>
3 <li [routerLinkActive]="['active']"><a [routerLink]="['/about']">About</a></li>
4</ul>
In this case, the active
class will be added to the <li>
element if the corresponding link is active.
- If you want to set the active class on an element without
[routerLink]
, you can use therouter.isActive()
method with the URL you want to check. For example:
1import { Router } from '@angular/router';
2
3class MyComponent {
4 constructor(private router: Router) {}
5
6 isRouteActive(route: string) {
7 return this.router.isActive(route, false);
8 }
9}
And in the HTML:
1<div *ngIf="isRouteActive('/home')">...</div>
- In Angular 8, you can use the
router.isActive()
method with the exact option set totrue
to check if a route is currently active. For example:
1import { Router } from '@angular/router';
2
3class MyComponent {
4 constructor(private router: Router) {}
5
6 isRouteActive(route: string) {
7 return this.router.isActive(route, true);
8 }
9}
And in the HTML:
1<div *ngIf="isRouteActive('/home')">...</div>
- In Angular 9+ (including Angular 10), you can simply use the
routerLinkActive
directive with theisActive
property to check if a route is currently active. For example:
1<a routerLink="/home" routerLinkActive #rla="routerLinkActive" [ngClass]="rla.isActive ? 'active' : 'inactive'">Home</a>
This way, the isActive
property of the routerLinkActive
directive will be added to the template variable rla
, and you can use it to determine if the current route is active.
相关文章推荐
- (heading level 1)
- Random Color Generator
- <html>
- 获取触发事件的元素的ID
- JavaScript中从URL中获取协议、域名和端口
- 在 JavaScript 中如何使用命名参数
- 在JavaScript中如何检查对象是否具有特定属性?
- 使用jQuery动态创建隐藏的表单元素
- 在Javascript中获取自Unix纪元以来的毫秒数
- 使用原生JavaScript选择具有"data-xxx"属性的所有元素(无需使用jQuery)
- 如何检查一个对象是否为日期对象?
- 在JavaScript中创建静态变量
- 使用JavaScript源映射(.map文件)
- 使用jQuery即时检测<input type="text">的所有更改
- <html>
- 在JavaScript中将数字转换为字符串的最佳方式
- 在JavaScript中执行整数除法和取余操作
- 如何获取当前日期和时间的秒数
- 在 Angular 中手动触发变更检测
- 在 JavaScript 中给今天的日期添加指定天数
- 将逗号分隔的字符串转换为数组的方法
- <!DOCTYPE html>
- +# 用JS解析HTML字符串
- 在Google Chrome中如何通过代码设置JavaScript断点
- 使用jQuery实现自动滚动到页面底部
- PHP中的shell_exec()和exec()命令的区别
- 在JavaScript中如何编写行内的IF语句
- "正确"的JSON日期格式是什么?
- 在JavaScript中给Date对象添加小时的方法
- 如何克隆一个 Date 对象?
- 使用 Fetch API 进行 GET 请求设置查询字符串
- 如何从JavaScript对象中删除项
- 阻止双击后文本选择的方法